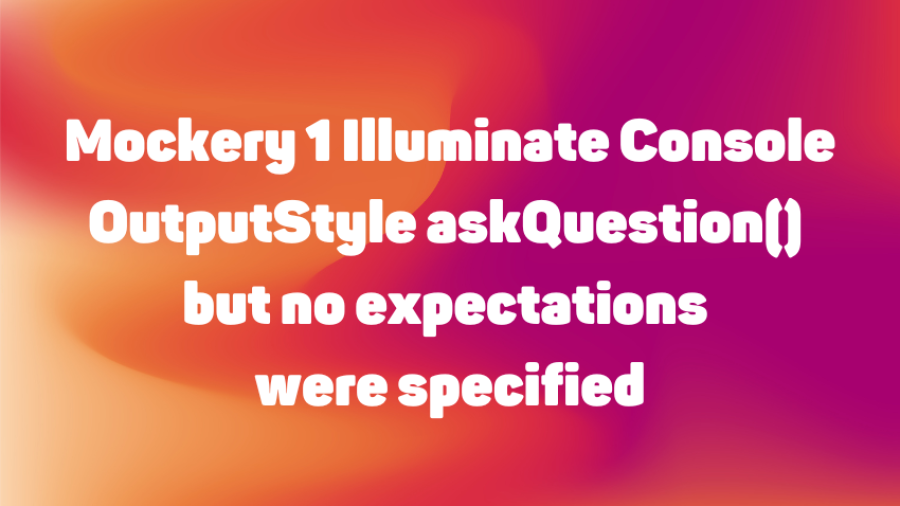
PestPHP is a testing framework with a focus on simplicity, in this post I'll explain how to use PestPHP within a Laravel package to test its functionality.
In order to test a Laravel package, a package called testbench is required. Testbench ready more about testbench at https://packages.tools/testbench/getting-started/introduction.html#installation
Install testbench and PestPHP package
composer require orchestra/testbench --dev
composer require pestphp/pest --dev
composer require pestphp/pest-plugin-laravel --dev
Inside composer.json autoload the tests directory I'm using Dcblogdev\\PackageName to represent the vendor username and repo name.
"autoload": {
"psr-4": {
"Dcblogdev\\PackageName\\": "src/",
"Dcblogdev\\PackageName\\Tests\\": "tests"
}
},
This will auto-load the tests directory at the root of the package.
Next, create a file called phpunit.xml.
<?xml version="1.0" encoding="UTF-8"?>
<phpunit
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
bootstrap="vendor/autoload.php"
backupGlobals="false"
backupStaticAttributes="false"
colors="true"
verbose="true"
convertErrorsToExceptions="true"
convertNoticesToExceptions="true"
convertWarningsToExceptions="true"
processIsolation="false"
stopOnFailure="false"
xsi:noNamespaceSchemaLocation="https://schema.phpunit.de/9.3/phpunit.xsd"
>
<coverage>
<include>
<directory suffix=".php">src/</directory>
</include>
</coverage>
<testsuites>
<testsuite name="Unit">
<directory suffix="Test.php">./tests/Unit</directory>
</testsuite>
</testsuites>
<php>
<env name="DB_CONNECTION" value="testing"/>
<env name="APP_KEY" value="base64:2fl+Ktvkfl+Fuz4Qp/A75G2RTiWVA/ZoKZvp6fiiM10="/>
</php>
</phpunit>
Here an APP_KEY is set, feel free to change this. Also, the Database connection is set to testing. This also sets the tests inside tests/Unit to be loaded. Add tests/Feature as needed.
Create a tests folder inside it create 2 files Pestp.php and TestCase.php
TestCase.php
<?php
namespace Dcblogdev\PackageName\Tests;
use Orchestra\Testbench\TestCase as Orchestra;
use Dcblogdev\PackageName\PackageNameServiceProvider;
class TestCase extends Orchestra
{
protected function getPackageProviders($app)
{
return [
PackageNameServiceProvider::class,
];
}
}
This loads the package service provider.
Pest.php
<?php
use Dcblogdev\PackageName\Tests\TestCase;
uses(TestCase::class)->in(__DIR__);
In Pest.php set any custom test helpers. This would load the TestCase and test in in the current tests directory using __DIR__ this allows pest to run in sub folders of tests ie Unit and Feature are the most common folders.
Next, create a folder called Unit inside tests and create a test file I'll use DemoTest.php
tests/Unit/DemoTest.php
test('confirm environment is set to testing', function () {
expect(config('app.env'))->toBe('testing');
});
No imports or classes are required. That's what's great about Pest you can concentrate on writing the test with minimal setup required.
From here you can write your tests using Pest in the same manner as you would writing PestPHP tests in a Laravel application.
Subscribe to my newsletter for the latest updates on my books and digital products.
Find posts, tutorials, and resources quickly.
Subscribe to my newsletter for the latest updates on my books and digital products.