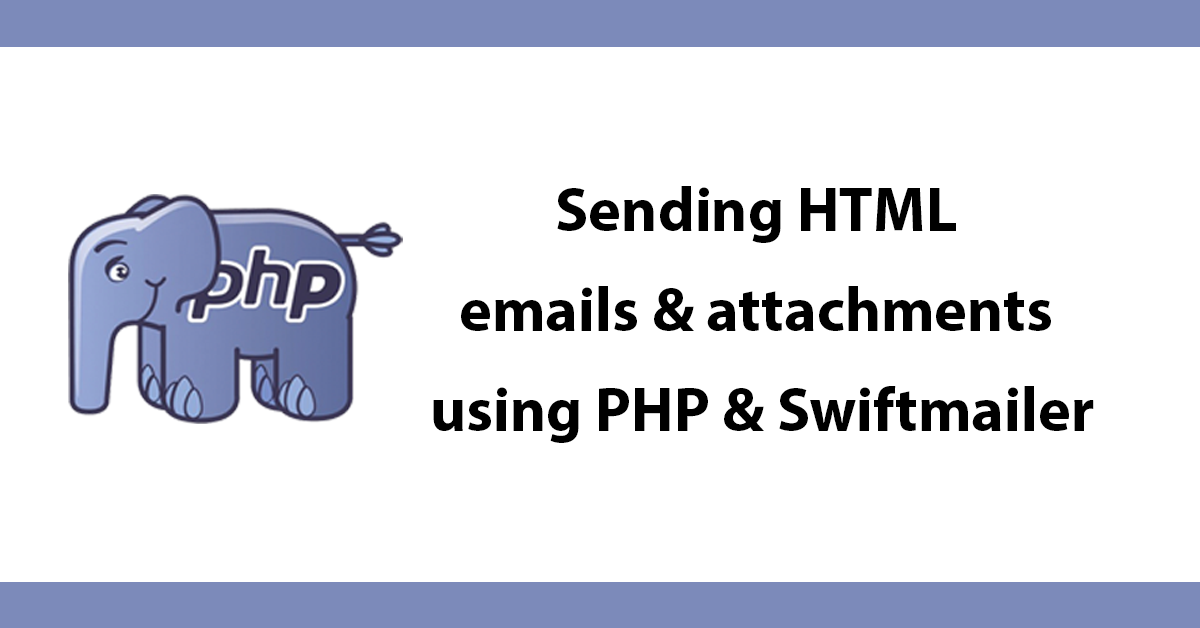
Laravel Framework Tutorials PHP & MySQL
Laravel makes downloading a server file very easy, in this post I'm going to show a way to download files but only let authenticated users.
First, a route group is used to set up the auth middleware:
Route::group(['middleware' => 'auth'], function () {
Next create a dedicated download route:
Route::get('download', function () {
Collect a request:
$path = request('f');
Detect the file extension
$extension = pathinfo($path, PATHINFO_EXTENSION);
Create a blocked list array, this is a list of all extensions we won't allow to be downloaded.
$blocked = ['php', 'htaccess'];
If the requested file is not in the blocked array then download it.
if (! in_array($extension, $blocked)) {
//download the file
return response()->download($path);
}
Putting it all together:
//only logged in users can download
Route::group(['middleware' => 'auth'], function () {
//respond to route /download?f=path
Route::get('download', function () {
//get the GET request
$path = request('f');
//look at the extension of the file being requested
$extension = pathinfo($path, PATHINFO_EXTENSION);
//create an array of items to reject being downloaded
$blocked = ['php', 'htaccess'];
//if the requested file is not in the blocked array
if (! in_array($extension, $blocked)) {
//download the file
return response()->download($path);
}
});
});
Subscribe to my newsletter for the latest updates on my books and digital products.
Find posts, tutorials, and resources quickly.
Subscribe to my newsletter for the latest updates on my books and digital products.