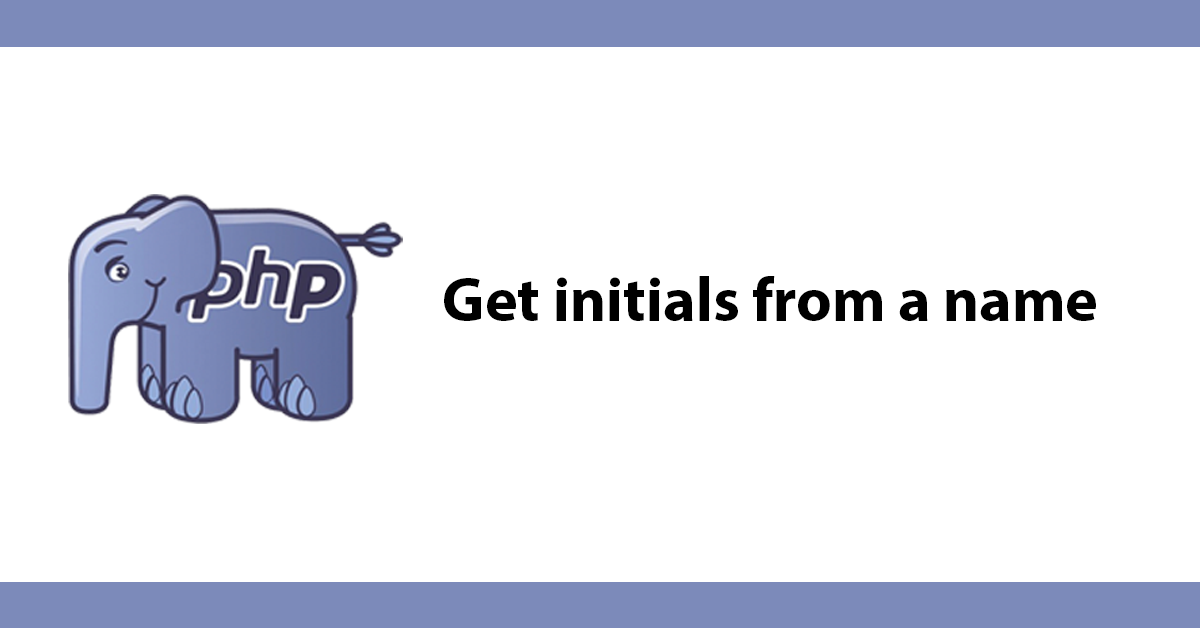
Development Tutorials PHP & MySQL Tools
Sending emails with raw PHP is very easy to do, but sending HTML email is a little more involved bring attachments into the equation and it can get very complex. Thankfully there are easy ways of sending mixed email types where you can send en email as HTML and also have a plain text version for email readers that do no support HTML and adding attachments is a simple case of pointing to the file to send!
I'm using a third party email library called Swiftmailer to use it download the files and upload the files inside the lib folder then your ready to use it.
There's a lot that can be done with swift check out their documentation for examples. Here is a code sample from their documentation on sending an email:
require_once 'lib/swift_required.php';
// Create the Transport
$transport = Swift_SmtpTransport::newInstance('smtp.example.org', 25)
->setUsername('your username')
->setPassword('your password')
;
/*
You could alternatively use a different transport such as Sendmail or Mail:
// Sendmail
$transport = Swift_SendmailTransport::newInstance('/usr/sbin/sendmail -bs');
// Mail
$transport = Swift_MailTransport::newInstance();
*/
// Create the Mailer using your created Transport
$mailer = Swift_Mailer::newInstance($transport);
// Create a message
$message = Swift_Message::newInstance('Wonderful Subject')
->setFrom(array('john@doe.com' => 'John Doe'))
->setTo(array('receiver@domain.org', 'other@domain.org' => 'A name'))
->setBody('Here is the message itself')
;
// Send the message
$result = $mailer->send($message);
That's not complicated but I prefer to have a small reusable helper function that can interface with the library with this function I can send out an HTML email with a small amount of code:
sendmail($to,$from,$subject,$textmail,$htmlmail);
With that call I pass in who I'm sending an email to the address I'm sending it from the subject and the 2 email versions plain text and html, to send an attachment I would reference the path to the file:
sendmail($to,$from,$subject,$textmail,$htmlmail,'pathtomyfile');
That's very reusable and simple to implement. The helper function is taken from the swift documentation with a few tweaks:
function sendmail($to,$from,$subject,$textmail,$htmlmail = NULL, $attachment = NULL){
require_once 'lib/swift_required.php';
//Mail
$transport = Swift_MailTransport::newInstance();
//Create the Mailer using your created Transport
$mailer = Swift_Mailer::newInstance($transport);
//Create the message
$message = Swift_Message::newInstance()
//Give the message a subject
->setSubject($subject)
//Set the From address with an associative array
->setFrom($from)
//Set the To addresses with an associative array
->setTo($to)
//Give it a body
->setBody($textmail);
if($htmlmail !=''){
//And optionally an alternative body
$message->addPart($htmlmail, 'text/html');
}
if($attachment !=''){
//Optionally add any attachments
$message->attach(
Swift_Attachment::fromPath($attachment)->setDisposition('inline')
);
}
//Send the message
$result = $mailer->send($message);
return $result;
}
The html part and attachments are optional, this make it very flexible perfect for sending fully formatted emails.
Subscribe to my newsletter for the latest updates on my books and digital products.
Find posts, tutorials, and resources quickly.
Subscribe to my newsletter for the latest updates on my books and digital products.