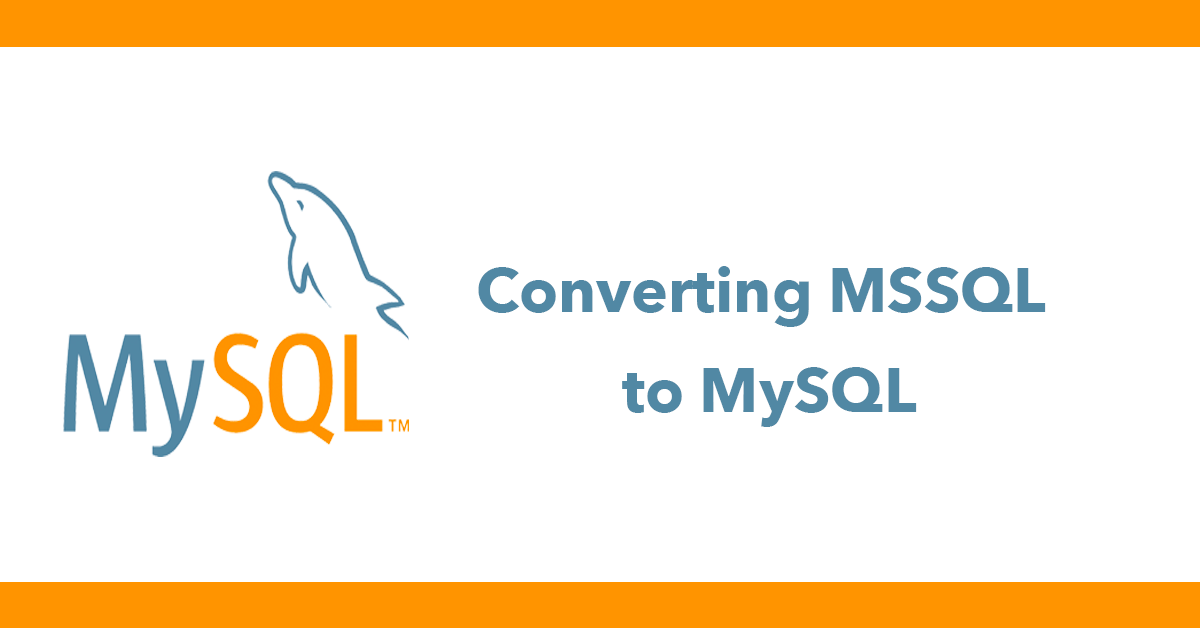
Development Tutorials PHP & MySQL
Sometimes you need to make improvements to your code and make things more efficient. Using some simple PHP Statistics can help you identify areas which need improving. These snippets offer useful insight into how efficiently your application is running.
So, for our first snippet we will look at script execution time. This will tell us how long it took the server to process and execute our PHP script. This is one of the most useful snippets. It would also be very useful for benchmarking PHP 5.6 versus 7.0.
To calculate the script execution time we need can make use of the $_SERVER super global. Since v5.4.0 $_SERVER[‘REQUEST_TIME_FLOAT’] will record the start time of your script. All we need to do now is declare microtime(true), microtome will return the current unix timestamp with microseconds. The boolean is set to true, which will return the value as a float so we can perform calculations with it. Now we need to at it to the end of our script and subtract it from the start time.
We will need a little formatting to make it a little easier to read. We will create a new variable which will store the time and then output it on our website.
<?php
//Get average CPU usage
$cpuUsage = sys_getloadavg();
echo "<h4><li><i class='fa fa-server'></i> CPU Load: <b>".$cpuUsage[0]."</b></li></h4>";
?>
Another useful statistic is the CPU load. This is a built in array in PHP, it will show the number of processes in the system run queue. The array will return three average samples for the last 1, 5 and 15 minutes respectively. This is a great way of seeing what kind of strain the server is under and how many thing it must process. This array would be useful for determining whether your server was almost at maximum capacity and would be fairly straightforward to set up some logic to limit any server downtime.
<?php
//Display server script execution time
$finishedTime = number_format((float)(microtime(true) - $_SERVER["REQUEST_TIME_FLOAT"]), 3, '.', ''); echo "<h4><li>Execution Time: <b>".$finishedTime." sec</b></li></h4>";
?>
With this snippet we will see the peak memory usage. We will have to values to look at here. The first is the used memory. This is the peak memory used for the script. Secondly, we have the peak memory allocated to the script. Using the two in conjunction give us some greater insight as to the internal working and efficiency of our code.
<?php
//Display Memory used and allocated
$usedMem = number_format((float) (memory_get_peak_usage(false) / 1024 / 1024), 2, '.', '');
echo "<li>Used Memory: <b>" . $usedMem . "MB</b></li>";
echo "<li><Allocated Memory: <b>" . (memory_get_peak_usage(true) / 1024 / 1024) . "MB</b></li>";
?>
Another useful thing would be to see the exact amount of database queries we are performing. This will allow us to trim the number and make our application leaner and faster. This snippet requires a little more setup than the last few, but the payoff is worth it.
I’m going to assume a MVC framework is being used here.
Firstly in out Database controller we create a new variable to store a count of all database queries. Afterwards in each method we add the following
<?php
//Display amount of PDO queries used
$queries = Database::getCount();
echo "<h4><li>PDO Queries: <b>44</b></li></h4>";
?>
This will increment the counter by one each time a method is called. Then we should create a method for the counter which we can call elsewhere which will simply return that number.
And finally we create a new variable to store the call to the DatabaseCount method which we can now use in our output.
/* Database Example */
<?php
class Database extends PDO
{
/**
* Create a variable to store the count of PDO Queries
*/
public static $queryCount = 0;
/**
* insert method
* @param string $table table name
* @param array $data array of columns and values
*/
public function insert($table, $data)
{
//Increment value of var for each PDO function in Helper
self::$queryCount++;
//Some code here...
}
public function getCount()
{
//Return the var
return self::$queryCount;
}
}
?>
So, after all of that you should have some useful PHP statistics now, which you can use to make code optimisations and improvements for speed, efficiency and just for some geeky stats if nothing else. These can really identify an areas of your application which may be lagging or slowing down your application. I hope you’ve had fun with these snippets.
Happy Coding!
Subscribe to my newsletter for the latest updates on my books and digital products.
Find posts, tutorials, and resources quickly.
Subscribe to my newsletter for the latest updates on my books and digital products.