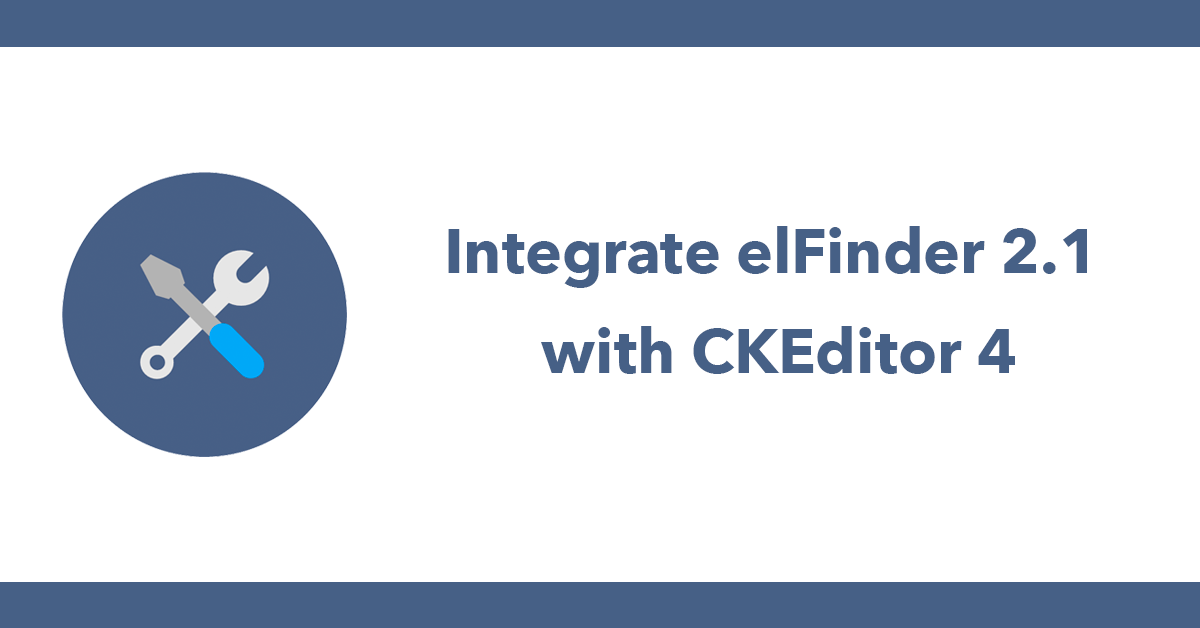
Tests Laravel Framework Tutorials PHPUnit
Laravel provides a range of methods to test parts of the framework, one of which is called blade() which allows you to test blade components work as expected.
Let's take a simple blade component:
@props([
'type' => 'submit'
])
<div>
<button type="{{ $type }}" {{ $attributes->merge(['class' => "btn btn-blue"]) }}>
{{ $slot }}
</button>
</div>
this creates a button with a type of submit by default which can be changed by using type="button" when calling the component:
<button type="button">Submit</button>
Create a test to confirm the button contains Submit text:
/** @test **/
public function button_can_submit(): void
{
$this->blade('<x-form.button>Submit</x-form.button>')
->assertSee('Submit');
}
The test passes since the button does contain Submit.
Next test the type can be changed from the default of submit to button:
/** @test **/
public function button_acts_as_a_button(): void
{
$this->blade('<x-button type="button">Submit</x-button>')
->assertSee('type="button"', false);
}
In order to test the HTML type="button" the assertSee method needs to stop escaping the markup, this is down by passing false as a second param.
As you can see testing blade components is simple to do, let's do a final test to test the class 'btn btn-blue' exists
/** @test **/
public function button_has_class(): void
{
$this->blade('<x-button>Submit</x-button>')
->assertSee('btn btn-blue');
}
Subscribe to my newsletter for the latest updates on my books and digital products.
Find posts, tutorials, and resources quickly.
Subscribe to my newsletter for the latest updates on my books and digital products.