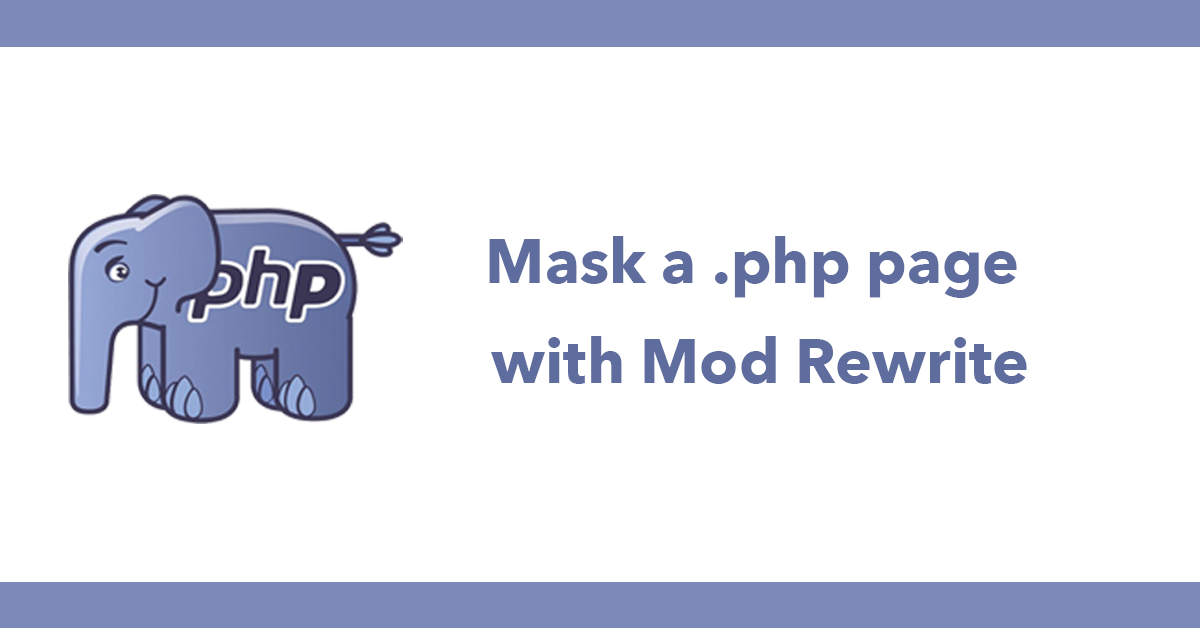
Outputting a forms contents to a CSV file is incredibly handy and easy to do, this post will cover the steps involved.
First create the form, for this post it will collect a name and email address.
<form action='' method='post'>
<p><label>Name</label><br><input type='text' name='name' value=''></p>
<p><label>Email</label><br><input type='text' name='email' value=''></p>
<p><input type='submit' name='submit' value='Submit'></p>
</form>
Next check if the form has been submitted.
if(isset($_POST['submit'])){
Then collect the data from the form.
$name = $_POST['name'];
$email = $_POST['email'];
Check if $name is empty if it is create an error array.
if($name ==''){
$error[] = 'Name is required';
}
Check for a valid email address, if not valid create an error array.
if(!filter_var($email, FILTER_VALIDATE_EMAIL)){
$error[] = 'Please enter a valid email address';
}
If no errors have been created then carry on
if(!isset($error)){
Next define the columns of the CSV file by comma separating the titles ending the row with a \n
$Content = "Name, Email\n";
Add the form data to the $Content, this will be the body of the CSV file.
$Content .= "$name, $email\n";
Next set the CSV filename and use headers to output the CSV file.
$FileName = "formdata-".date("d-m-y-h:i:s").".csv";
header('Content-Type: application/csv');
header('Content-Disposition: attachment; filename="' . $FileName . '"');
echo $Content;
exit();
If their are errors display them
if(isset($error)){
foreach($error as $error){
echo "<p style='color:#ff0000'>$error</p>";
}
}
<?php
if(isset($_POST['submit'])){
//collect form data
$name = $_POST['name'];
$email = $_POST['email'];
//check name is set
if($name ==''){
$error[] = 'Name is required';
}
//check for a valid email address
if(!filter_var($email, FILTER_VALIDATE_EMAIL)){
$error[] = 'Please enter a valid email address';
}
//if no errors carry on
if(!isset($error)){
# Title of the CSV
$Content = "Name, Email\n";
//set the data of the CSV
$Content .= "$name, $email\n";
# set the file name and create CSV file
$FileName = "formdata-".date("d-m-y-h:i:s").".csv";
header('Content-Type: application/csv');
header('Content-Disposition: attachment; filename="' . $FileName . '"');
echo $Content;
exit();
}
}
//if their are errors display them
if(isset($error)){
foreach($error as $error){
echo "<p style='color:#ff0000'>$error</p>";
}
}
?>
<form action='' method='post'>
<p><label>Name</label><br><input type='text' name='name' value=''></p>
<p><label>Email</label><br><input type='text' name='email' value=''></p>
<p><input type='submit' name='submit' value='Submit'></p>
</form>
Subscribe to my newsletter for the latest updates on my books and digital products.
Find posts, tutorials, and resources quickly.
Subscribe to my newsletter for the latest updates on my books and digital products.