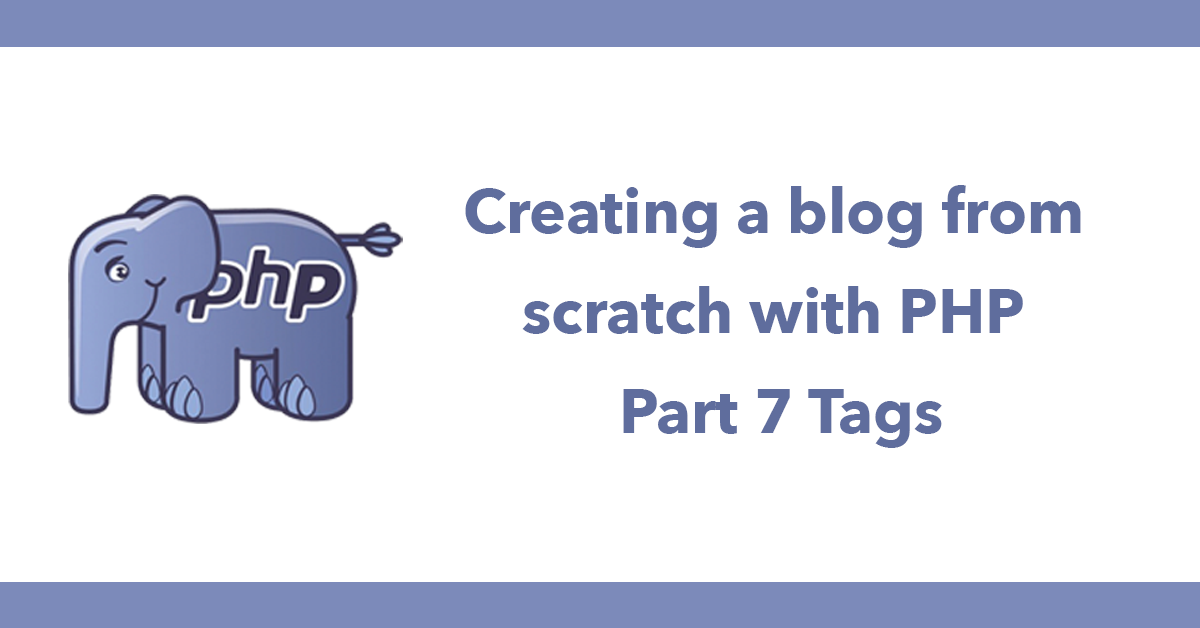
PHP's in_array is useful to determine if an item is in an array but when needing to compare multiple values against in_array no results will be found.
A way round this is to loop through the values and compare each one in turn, this function is pefect that that task:
function isInArray($needle, $haystack)
{
foreach ($needle as $stack) {
if (in_array($stack, $haystack)) {
return true;
}
}
return false;
}
This function expects 2 arrays to be passed, it will then loop through the keys and compare them. If no matches are found false is returned.
Here are two arrays, the exclude array is a list of people to exclude.
$people = array(
'Dave',
'Emma',
'Terry',
'Cath'
);
$exclude = array(
'emma'
);
Using array_map we can make all items lowercase so case sensativity does not get in the way.
$people = array_map('strtolower', $people);
$exclude = array_map('strtolower', $exclude);
Check if any person from the excludes array is in the people array.
if(isInArray($exclude, $people) == true){
echo 'people from excludes are in the array $people';
} else {
echo 'no exclusions';
}
function isInArray($needle, $haystack)
{
foreach ($needle as $stack) {
if (in_array($stack, $haystack)) {
return true;
}
}
return false;
}
$people = array(
'Dave',
'Emma',
'Terry',
'Cath'
);
$exclude = array(
'emma'
);
$people = array_map('strtolower', $people);
$exclude = array_map('strtolower', $exclude);
if(isInArray($exclude, $people) == true){
echo 'people from excludes are in the array $people';
} else {
echo 'no exclusions';
}
Subscribe to my newsletter for the latest updates on my books and digital products.
Find posts, tutorials, and resources quickly.
Subscribe to my newsletter for the latest updates on my books and digital products.