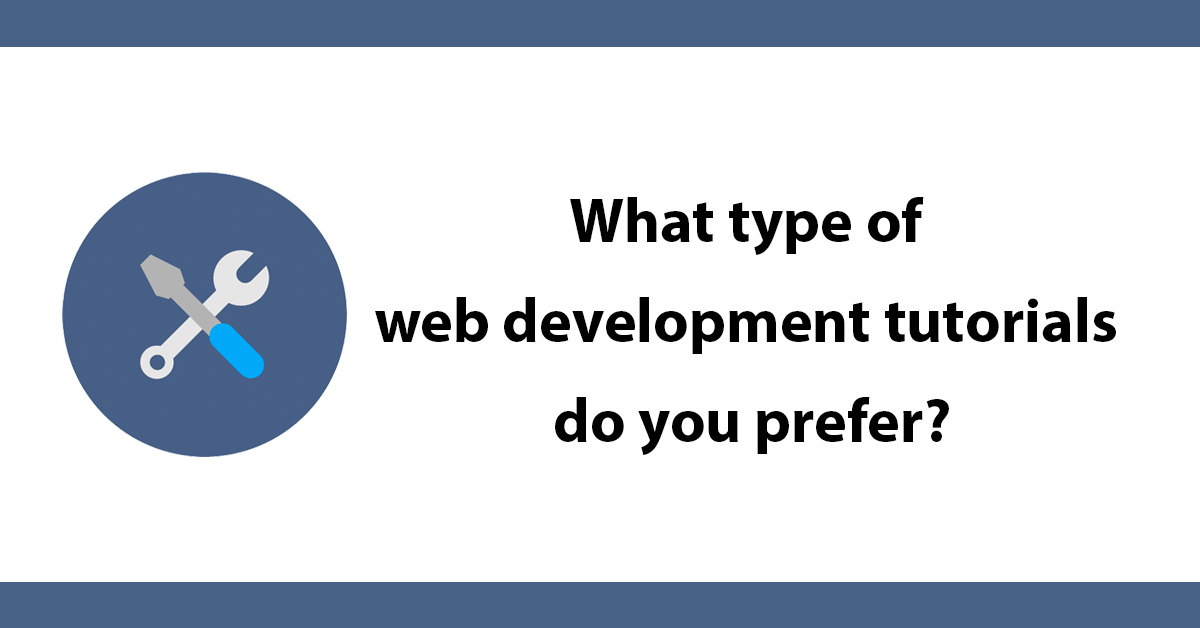
In this tutorial, I’m building a calculator that works out the gross profit percent, gross margin percent and the profit from a sell and unit price. Going another step further I’ll have a form that will automatically calculate these as soon as the sell and buy price has been inputted using jQuery.
Sample data:
sell price = 75
buy price = 40
profit = 35 (Sell price – Buy price)
The Gross Profit is the profit divided by the sell price times 100 so the formula is:
profit / sell price * 100
(In this example using the figures above)
gross profit = 35/75*100
The margin is 100 times sell price minus the buy price divided by the buy price so the formula is
100 * (sell price - buy price) / price cost
(In this example using the figures above)
gross margin = 100 * (75 - 40) / 40
<table>
<tr>
<th>Sell Price £</th>
<th>Buy Price £</th>
<th>Gross Profit %</th>
<th>Gross Margin %</th>
<th>Profit £</th>
</tr>
<tr>
<td><input class='item sellprice' type='number' value='0'></td>
<td><input class='item buyprice' type='number' value='0'></td>
<td><input class='grossProfit' readonly type='number' value='0'></td>
<td><input class='grossMargin' readonly type='number' value='0'></td>
<td><input class='profit' readonly type='number' value='0'></td>
</tr>
</table>
this is a standard table but a few important points to mention:
<script type="text/javascript" src="jquery.js"></script>
<script type="text/javascript">
$(function() {
$(document).on("change", ".item", function () {
var sellprice = $('.sellprice').val();
var buyprice = $('.buyprice').val();
if (sellprice > 0 && buyprice > 0) {
var profit = sellprice - buyprice;
var grossProfit = profit / buyprice * 100;
var grossMargin = 100 * (sellprice - buyprice) / sellprice;
$('.grossProfit').val(grossProfit.toFixed(2));
$('.grossMargin').val(grossMargin.toFixed(2));
$('.profit').val(profit);
}
});
});
</script>
Create a trigger that listens for changes, by using the change event on the document, this will then trigger the closure to run when there is a change on either the sell or buy price since they both have a class of item.
Create variables that read directly from the input for both sell and buy price.
Next, as long as both sell and buy price is more than 0 run the calculations.
Setup the 3 calculations passing in the variables, lastly add the totals of the calculations to the inputs append .toFixed(2) to round the decimals to only 2 digits.
That’s it, with this setup after entering a buy and sell price the gross profit percent, gross margin percent and profit will be calculated and displayed in the inputs.
Subscribe to my newsletter for the latest updates on my books and digital products.
Find posts, tutorials, and resources quickly.
Subscribe to my newsletter for the latest updates on my books and digital products.