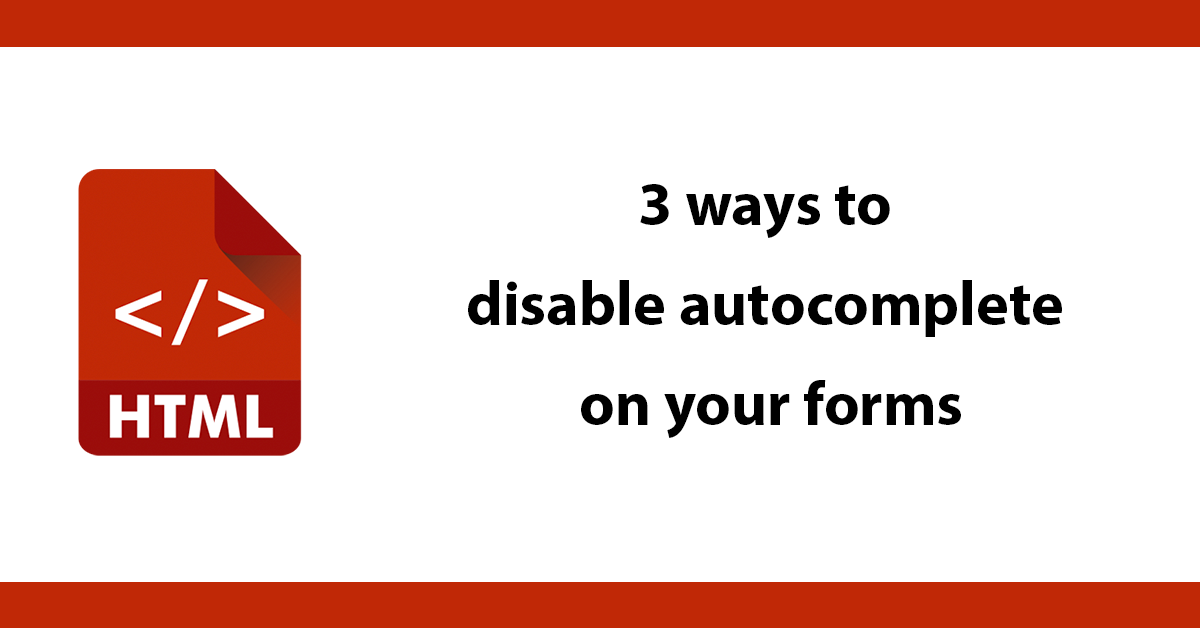
This tutorial will show you how to upload an image and create 2 images, a full sized image and a thumbnail then delete the uploaded image from the server as it's no longer needed.
As the image paths will be stored in a database you'll need to connect to your database and also have a table to store the image in. Create the following table as we'll be using this structure to store the image paths, title and description.
the usual database connection:
$dbhost = 'localhost';
$dbuser = 'database username';
$dbpass = 'password';
$dbname = 'database username';
// make a connection to mysql here
$conn = mysql_connect ($dbhost, $dbuser, $dbpass) or die ("I cannot connect to the database because: " . mysql_error());
mysql_select_db ($dbname) or die ("I cannot select the database '$dbname' because: " . mysql_error());
mysql_query("CREATE TABLE gal_images (
imageID INT(4) NOT NULL AUTO_INCREMENT,
imageTitle VARCHAR(255) NOT NULL,
imageThumb VARCHAR(255) NOT NULL,
imageFull VARCHAR(255) NOT NULL,
imageDesc TEXT NULL,
PRIMARY KEY(imageID))")or die(mysql_error());
Next we create a simple form for a title the image and a description. to enable image to be uploaded we need to add enctype="multipart/form-data" to the form otherwise it won't work no matter what you try.
<form enctype="multipart/form-data" action="" method="post">
<fieldset>
<legend>Upload Picture</legend>
<p>Only JPEG, GIF, or PNG images accepted</p>
<p><label>Title<br /></label><input type="text" name="imageTitle" <?php if (isset($error)){ echo "value="$imageTitle""; }?> /></p>
<p><label>Image<br /></label><input type="file" name="uploaded" /></p>
<p><label>Description<br /></label><textarea name="imageDesc" cols="50" rows="10"><?php if (isset($error)){ echo "$imageDesc"; }?></textarea></p>
<p><label> </label><input type="submit" name="submit" value="Add Image" /></p>
</fieldset>
</form>
The following code will only run if the form has been submitted by checking if isset then pass the name of the submit button, next check are done to make sure the title and description is not empty and an image has been uploaded, otherwise an error is generated that will be used later.
// if form submitted then process form
if (isset($_POST['submit'])){
// check feilds are not empty
$imageTitle = trim($_POST['imageTitle']);
if (strlen($imageTitle) < 3 || strlen($imageTitle) > 255) {
$error[] = 'Title must be at between 3 and 255 charactors.';
}
// check fields are not empty or check image file size
if (!isset($_FILES["uploaded"])) {
$error[] = 'You forgot to select an image.';
}
// check fields are not empty
$imageDesc = trim($_POST['imageDesc']);
if (strlen($imageDesc) < 3) {
$error[] = 'Please enter a description for your image.';
}
Next set a location to upload the image to, this will only be stored here while we make the 2 new images then it will be deleted, make sure the path is relative to the script. Then check the image type by passing $_FILES["uploaded"]["type"] to a switch statement to determine the image type.
// location where initial upload will be moved to
$target = "images/gallery/" . $_FILES['uploaded']['name'] ;
// find the type of image
switch ($_FILES["uploaded"]["type"]) {
case $_FILES["uploaded"]["type"] == "image/gif":
move_uploaded_file($_FILES["uploaded"]["tmp_name"],$target);
break;
case $_FILES["uploaded"]["type"] == "image/jpeg":
move_uploaded_file($_FILES["uploaded"]["tmp_name"],$target);
break;
case $_FILES["uploaded"]["type"] == "image/pjpeg":
move_uploaded_file($_FILES["uploaded"]["tmp_name"],$target);
break;
case $_FILES["uploaded"]["type"] == "image/png":
move_uploaded_file($_FILES["uploaded"]["tmp_name"],$target);
break;
case $_FILES["uploaded"]["type"] == "image/x-png":
move_uploaded_file($_FILES["uploaded"]["tmp_name"],$target);
break;
default:
$error[] = 'Wrong image type selected. Only JPG, PNG or GIF accepted!.';
}
If no errors have been created the next step is to clean up the form data
// if validation is okay then carry on
if (!$error) {
// post form data
$imageTitle = $_POST['imageTitle'];
$imageDesc = $_POST['imageDesc'];
//strip any tags from input
$imageTitle = strip_tags($imageTitle);
$imageDesc = strip_tags($imageDesc);
// add slashes if needed
if(!get_magic_quotes_gpc())
{
$imageTitle = addslashes($imageTitle);
$imageDesc = addslashes($imageDesc);
}
// remove any harhful code and stop sql injection
$imageTitle = mysql_real_escape_string($imageTitle);
$imageDesc = mysql_real_escape_string($imageDesc);
Now set 2 file paths the first (dst_filename_full) for the location to store the full sized image and the second to store the thumbnail. I've chosen to keep these in separate folder to keep things clean (don't forget this folders need to exist for this to work)
Next we call a function to make a full sized image createthumbfull() pass the function the uploaded file ($src_filename) and the destination path ($dst_filename_full) this will make a new image and save it to your server, the image will have a random filename.
Next insert the title image paths and description data to a database, $thumb_Add_thumb and $thumb_Add_full come from the function calls via a global command (see comments inside the function further down the page). Notice the command unlink ($src_filename); this removes the image from the server as we no longer needed it.
//add target location to varible $src_filename
$src_filename = $target;
// define file locations for full sized and thumbnail images
$dst_filename_full = 'images/gallery/full/';
$dst_filename_thumb = 'images/gallery/thumb/';
// create the images
createthumbfull($src_filename, $dst_filename_full); //call function to create full sized image
createthumb($src_filename, $dst_filename_thumb); //call function to create thumbnail image
// delete original image as its not needed any more.
unlink ($src_filename);
// insert data into images table
$query = "INSERT INTO gal_images (imageTitle, imageThumb, imageFull, imageDesc) VALUES
('$imageTitle', '$thumb_Add_thumb', '$thumb_Add_full', '$imageDesc')";
$result = mysql_query($query) or die ('Cannot add image because: '. mysql_error());
// show a message to confirm results
echo "<h3 align='center'> $imageTitle uploaded</h3>";
To show any errors if there have been any call an error function above the form:
//dispaly any errors
errors($error);
All functions should be stored in a functions file to keep your code organised but for the sake of this tutorial all the functions are in the same file.
This error function counts any errors that are stored in an array and loops through them and displays them.
function errors($error){
if (!empty($error))
{
$i = 0;
echo "<blockquote>n";
while ($i < count($error)){
echo "<p><span class="warning">".$error[$i]."</span></p>n";
$i ++;}
echo "</blockquote>n";
}// close if empty errors
} // close function
Next the function to make a full sized image I've placed comments throughout the function to see show what does what, the height is automatic the inly thing you need to set in this function is the desired width I've set it to 600.
function createthumbfull($src_filename, $dst_filename_full)
{
// Get information about the image
list($src_width, $src_height, $type, $attr) = getimagesize( $src_filename );
// Load the image based on filetype
switch( $type ) {
case IMAGETYPE_JPEG:
$starting_image = imagecreatefromjpeg( $src_filename );
break;
case IMAGETYPE_PNG:
$starting_image = imagecreatefrompng( $src_filename );
break;
case IMAGETYPE_GIF:
$starting_image = imagecreatefromgif( $src_filename );
break;
default:
return false;
}
// get the image to create thumbnail from
$starting_image;
// get image width
$width = imagesx($starting_image);
// get image height
$height = imagesy($starting_image);
// size to create thumnail width
$thumb_width = 600;
// divide iwidth by specified thumb size
$constant = $width/$thumb_width;
// round height by constant and add t thumb_height
$thumb_height = round($height/$constant, 0);
//create thumb with true colours
$thumb_image = imagecreatetruecolor($thumb_width, $thumb_height);
//create thumbnail resampled to make image smooth
imagecopyresampled($thumb_image, $starting_image, 0, 0, 0, 0, $thumb_width, $thumb_height, $width, $height);
$ran = rand () ;
$thumb1 = $ran.".jpg";
global $thumb_Add_full;
$thumb_Add_full = $dst_filename_full;
$thumb_Add_full .= $thumb1;
imagejpeg($thumb_image, "" .$dst_filename_full. "$thumb1");
}
This next function create the thumbnail version, to adjust the width and height of the thumbnail adjust the sizes accordingly.
function createthumb($src_filename, $dst_filename_thumb)
{
$size = getimagesize($src_filename); // get image size
$stype = $size['mime']; //get image type : gif,png,jpg
$w = $size[0]; // width of image
$h = $size[1]; // height of image
//do a switch statment to create image from right image type
switch($stype) {
//if image is gif create from gif
case 'image/gif':
$simg = imagecreatefromgif($src_filename);
break;
//if image is jpeg create from jpeg
case 'image/jpeg':
$simg = imagecreatefromjpeg($src_filename);
break;
//if image is png create from png
case 'image/png':
$simg = imagecreatefrompng($src_filename);
break;
}
$width = $w; // get image width
$height = $h; // get image height
// size to create thumnail width
$thumb_width = 150;
$thumb_height = 150;
//use true colour for image
$dimg = imagecreatetruecolor($thumb_width, $thumb_height);
$wm = $width/$thumb_width; //width divided by new width
$hm = $height/$thumb_height; //height divided by new height
$h_height = $thumb_height/2; //ass new height and chop in half
$w_height = $thumb_width/2; //ass new height and chop in half
//if original width is more then original height then modify by width
if($w> $h) {
$adjusted_width = $w / $hm; // add original width and divide it by modified height and add to var
$half_width = $adjusted_width / 2; // chop width in half
$int_width = $half_width - $w_height; // take away modified height from new width
//make a copy of the image
imagecopyresampled($dimg,$simg,-$int_width,0,0,0,$adjusted_width,$thumb_height,$w,$h);
//else if original width is less or equal to original height then modify by height
} elseif(($w <$h) || ($w == $h)) {
$adjusted_height = $h / $wm; // diving original height by modified width
$half_height = $adjusted_height / 2; // chop height in half
$int_height = $half_height - $h_height; // take away modified height from new width
//make a copy of the image
imagecopyresampled($dimg,$simg,0,-$int_height,0,0,$thumb_width,$adjusted_height,$w,$h);
} else { // don't modify image and make a copy
imagecopyresampled($dimg,$simg,0,0,0,0,$thumb_width,$thumb_height,$w,$h);
}
$ran = "thumb_".rand (); // generate random number and add to a string
$thumb2 = $ran.".jpg"; //add random string to a var and append .jpg on the end
global $thumb_Add_thumb; //make this var available outside the function as it will be needed later.
$thumb_Add_thumb = $dst_filename_thumb; // add destination
$thumb_Add_thumb .= $thumb2; // append file name on the end
//actually create the final image
imagejpeg($dimg, "" .$dst_filename_thumb. "$thumb2");
}
That's everything you need to create thumbnails from an uploaded form, to illustrate the process better I've put the full script below:
<?php
//database properties
$dbhost = 'localhost';
$dbuser = 'database username';
$dbpass = 'password';
$dbname = 'database username';
// make a connection to mysql here
$conn = mysql_connect ($dbhost, $dbuser, $dbpass) or die ("I cannot connect to the database because: " . mysql_error());
mysql_select_db ($dbname) or die ("I cannot select the database '$dbname' because: " . mysql_error());
/******** start function ********/
function errors($error){
if (!empty($error))
{
$i = 0;
echo "<blockquote>n";
while ($i < count($error)){
echo "<p><span class="warning">".$error[$i]."</span></p>n";
$i ++;}
echo "</blockquote>n";
}// close if empty errors
} // close function
// ----------------Create Smaller version of large image---------------------
function createthumbfull($src_filename, $dst_filename_full)
{
// Get information about the image
list($src_width, $src_height, $type, $attr) = getimagesize( $src_filename );
// Load the image based on filetype
switch( $type ) {
case IMAGETYPE_JPEG:
$starting_image = imagecreatefromjpeg( $src_filename );
break;
case IMAGETYPE_PNG:
$starting_image = imagecreatefrompng( $src_filename );
break;
case IMAGETYPE_GIF:
$starting_image = imagecreatefromgif( $src_filename );
break;
default:
return false;
}
// get the image to create thumbnail from
$starting_image;
// get image width
$width = imagesx($starting_image);
// get image height
$height = imagesy($starting_image);
// size to create thumnail width
$thumb_width = 600;
// divide iwidth by specified thumb size
$constant = $width/$thumb_width;
// round height by constant and add t thumb_height
$thumb_height = round($height/$constant, 0);
//create thumb with true colours
$thumb_image = imagecreatetruecolor($thumb_width, $thumb_height);
//create thumbnail resampled to make image smooth
imagecopyresampled($thumb_image, $starting_image, 0, 0, 0, 0, $thumb_width, $thumb_height, $width, $height);
$ran = rand () ;
$thumb1 = $ran.".jpg";
global $thumb_Add_full;
$thumb_Add_full = $dst_filename_full;
$thumb_Add_full .= $thumb1;
imagejpeg($thumb_image, "" .$dst_filename_full. "$thumb1");
}
//-------------------- create thumbnail --------------------------------------------
//$src_filename = source of image
//$dst_filename_thumb = location to store final image
function createthumb($src_filename, $dst_filename_thumb)
{
$size = getimagesize($src_filename); // get image size
$stype = $size['mime']; //get image type : gif,png,jpg
$w = $size[0]; // width of image
$h = $size[1]; // height of image
//do a switch statment to create image from right image type
switch($stype) {
//if image is gif create from gif
case 'image/gif':
$simg = imagecreatefromgif($src_filename);
break;
//if image is jpeg create from jpeg
case 'image/jpeg':
$simg = imagecreatefromjpeg($src_filename);
break;
//if image is png create from png
case 'image/png':
$simg = imagecreatefrompng($src_filename);
break;
}
$width = $w; // get image width
$height = $h; // get image height
// size to create thumnail width
$thumb_width = 150;
$thumb_height = 150;
//use true colour for image
$dimg = imagecreatetruecolor($thumb_width, $thumb_height);
$wm = $width/$thumb_width; //width divided by new width
$hm = $height/$thumb_height; //height divided by new height
$h_height = $thumb_height/2; //ass new height and chop in half
$w_height = $thumb_width/2; //ass new height and chop in half
//if original width is more then original height then modify by width
if($w> $h) {
$adjusted_width = $w / $hm; // add original width and divide it by modified height and add to var
$half_width = $adjusted_width / 2; // chop width in half
$int_width = $half_width - $w_height; // take away modified height from new width
//make a copy of the image
imagecopyresampled($dimg,$simg,-$int_width,0,0,0,$adjusted_width,$thumb_height,$w,$h);
//else if original width is less or equal to original height then modify by height
} elseif(($w <$h) || ($w == $h)) {
$adjusted_height = $h / $wm; // diving original height by modified width
$half_height = $adjusted_height / 2; // chop height in half
$int_height = $half_height - $h_height; // take away modified height from new width
//make a copy of the image
imagecopyresampled($dimg,$simg,0,-$int_height,0,0,$thumb_width,$adjusted_height,$w,$h);
} else { // don't modify image and make a copy
imagecopyresampled($dimg,$simg,0,0,0,0,$thumb_width,$thumb_height,$w,$h);
}
$ran = "thumb_".rand (); // generate random number and add to a string
$thumb2 = $ran.".jpg"; //add random string to a var and append .jpg on the end
global $thumb_Add_thumb; //make this var available outside the function as it will be needed later.
$thumb_Add_thumb = $dst_filename_thumb; // add destination
$thumb_Add_thumb .= $thumb2; // append file name on the end
//actually create the final image
imagejpeg($dimg, "" .$dst_filename_thumb. "$thumb2");
}
/********end functions ***********/
// if form submitted then process form
if (isset($_POST['submit'])){
// check feilds are not empty
$imageTitle = trim($_POST['imageTitle']);
if (strlen($imageTitle) < 3 || strlen($imageTitle) > 255) {
$error[] = 'Title must be at between 3 and 255 charactors.';
}
// check feilds are not empty or check image file size
if (!isset($_FILES["uploaded"])) {
$error[] = 'You forgot to select an image.';
}
// check feilds are not empty
$imageDesc = trim($_POST['imageDesc']);
if (strlen($imageDesc) < 3) {
$error[] = 'Please enter a description for your image.';
}
// location where inital upload will be moved to
$target = "images/gallery/" . $_FILES['uploaded']['name'] ;
// find the type of image
switch ($_FILES["uploaded"]["type"]) {
case $_FILES["uploaded"]["type"] == "image/gif":
move_uploaded_file($_FILES["uploaded"]["tmp_name"],$target);
break;
case $_FILES["uploaded"]["type"] == "image/jpeg":
move_uploaded_file($_FILES["uploaded"]["tmp_name"],$target);
break;
case $_FILES["uploaded"]["type"] == "image/pjpeg":
move_uploaded_file($_FILES["uploaded"]["tmp_name"],$target);
break;
case $_FILES["uploaded"]["type"] == "image/png":
move_uploaded_file($_FILES["uploaded"]["tmp_name"],$target);
break;
case $_FILES["uploaded"]["type"] == "image/x-png":
move_uploaded_file($_FILES["uploaded"]["tmp_name"],$target);
break;
default:
$error[] = 'Wrong image type selected. Only JPG, PNG or GIF accepted!.';
}
// if valadation is okay then carry on
if (!$error) {
// post form data
$imageTitle = $_POST['imageTitle'];
$imageDesc = $_POST['imageDesc'];
//strip any tags from input
$imageTitle = strip_tags($imageTitle);
$imageDesc = strip_tags($imageDesc);
// add slashes if needed
if(!get_magic_quotes_gpc())
{
$imageTitle = addslashes($imageTitle);
$imageDesc = addslashes($imageDesc);
}
// remove any harhful code and stop sql injection
$imageTitle = mysql_real_escape_string($imageTitle);
$imageDesc = mysql_real_escape_string($imageDesc);
//add target location to varible $src_filename
$src_filename = $target;
// define file locations for full sized and thumbnail images
$dst_filename_full = 'images/gallery/full/';
$dst_filename_thumb = 'images/gallery/thumb/';
// create the images
createthumbfull($src_filename, $dst_filename_full); //call function to create full sized image
createthumb($src_filename, $dst_filename_thumb); //call function to create thumbnail image
// delete original image as its not needed any more.
unlink ($src_filename);
// insert data into images table
$query = "INSERT INTO gal_images (imageTitle, imageThumb, imageFull, imageDesc) VALUES
('$imageTitle', '$thumb_Add_thumb', '$thumb_Add_full', '$imageDesc')";
$result = mysql_query($query) or die ('Cannot add image because: '. mysql_error());
// show a message to confirm results
echo "<h3 align='center'> $imageTitle uploaded</h3>";
}
}
//dispaly any errors
errors($error);
?>
<form enctype="multipart/form-data" action="" method="post">
<fieldset>
<legend>Upload Picture</legend>
<p>Only JPEG, GIF, or PNG images accepted</p>
<p><label>Title<br /></label><input type="text" name="imageTitle" <?php if (isset($error)){ echo "value="$imageTitle""; }?> /></p>
<p><label>Image<br /></label><input type="file" name="uploaded" /></p>
<p><label>Description<br /></label><textarea name="imageDesc" cols="50" rows="10"><?php if (isset($error)){ echo "$imageDesc"; }?></textarea></p>
<p><label> </label><input type="submit" name="submit" value="Add Image" /></p>
</fieldset>
</form>
Subscribe to my newsletter for the latest updates on my books and digital products.
Find posts, tutorials, and resources quickly.
Subscribe to my newsletter for the latest updates on my books and digital products.