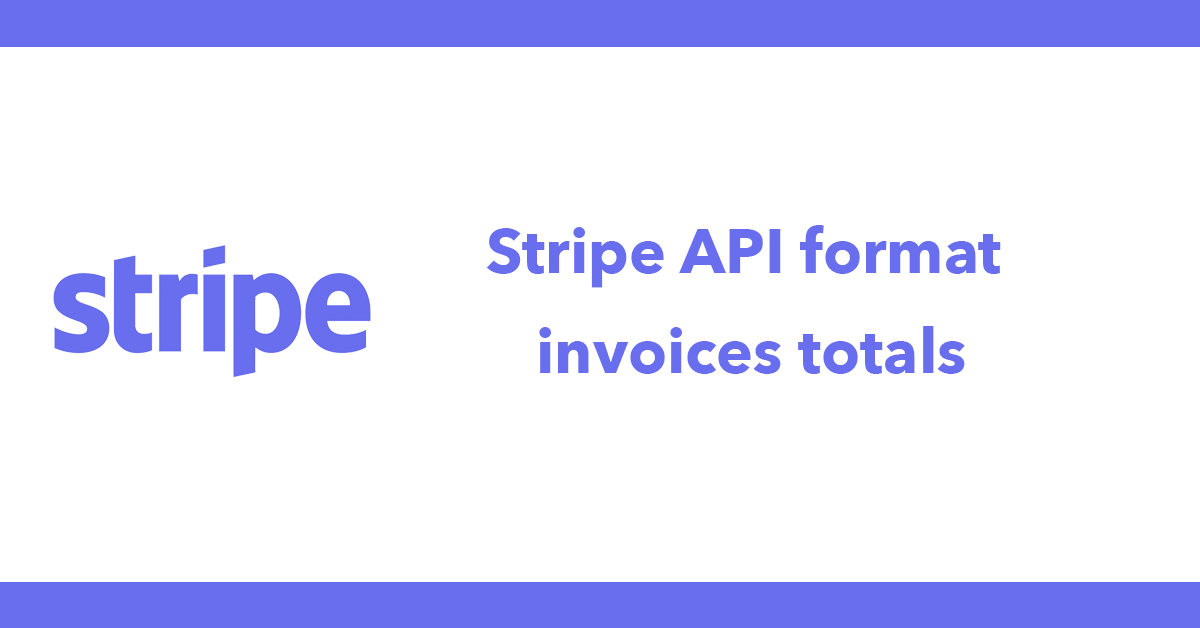
Using the YouTube API its possible to upload videos to your own YouTube account using their API, this tutorial explains the steps necessary to accomplish this.
This tutorial is based on the guide available from https://developers.google.com/youtube/2.0/developers_guide_php That guide is very informative and covers a lot in detail, I did find it a little difficult to follow, my aim is to write a more compact route.
In order to use the YouTube API you will need to download the Zend_Gdata library from Zend http://framework.zend.com/downloads/latest You will also need a YouTube Developer Key in order to authenticate and upload videos, one can be crated at https://code.google.com/apis/youtube/dashboard
First step is turn on session_start as sessions will be required also include the zend library files.
<?php
session_start();
require_once 'Zend/Loader.php'; // the Zend dir must be in your include_path
Zend_Loader::loadClass('Zend_Gdata_YouTube');
Zend_Loader::loadClass('Zend_Gdata_AuthSub');
</pre>
<p>The following function is required for making a link to YouTube to request a token</p>
<pre lang="php">
function getAuthSubRequestUrl()
{
$next = 'http://example.com/your-youtube-upload';
$scope = 'http://gdata.youtube.com';
$secure = false;
$session = true;
return Zend_Gdata_AuthSub::getAuthSubTokenUri($next, $scope, $secure, $session);
}
Next a check is made, if there is no session of sessionToken and a GET request for token does not exists then create a link to request one, once the request has been made save that to a session.
//generate link if no session or token has been requested
if (!isset($_SESSION['sessionToken']) && !isset($_GET['token']) ){
echo '<a href="' . getAuthSubRequestUrl() . '">Login to YouTube API!</a>';
//if token has been requested but not saved to a session then save the new token to a session
} else if (!isset($_SESSION['sessionToken']) && isset($_GET['token'])) {
$_SESSION['sessionToken'] = Zend_Gdata_AuthSub::getAuthSubSessionToken($_GET['token']);
}
Next an authorisation request will be made, in order for this to work the sessionToken must exist or an error will be created to avoid this an if statement is used so only if the session exists will a request be made.
if(isset($_SESSION['sessionToken'])) {
Next create a YouTube object by calling the method Zend_Gdata_YouTube this method expects four parameters, an httpClient, developers key, clientID and application id.
The clientID is no longer required so pass in NULL in its place. Make sure the developerKey is set to your own key.
$httpClient = Zend_Gdata_AuthSub::getHttpClient($_SESSION['sessionToken']);
$developerKey = 'you-developer-key-here';
$applicationId = 'Demo Application';
$yt = new Zend_Gdata_YouTube($httpClient, $applicationId, NULL, $developerKey);
Next create a video object that will contain the title,description and category for the uploaded video.
$myVideoEntry = new Zend_Gdata_YouTube_VideoEntry();
$myVideoEntry->setVideoTitle('My Test Movie');
$myVideoEntry->setVideoDescription('My Test Movie');
$myVideoEntry->setVideoCategory('Autos'); // The category must be a valid YouTube category!
The next variables are used to create the path to YouTube for submitting the video on submitting the form and setting a redirect url after the submitted video has finished, this should be the url of your application.
$tokenHandlerUrl = 'http://gdata.youtube.com/action/GetUploadToken';
$tokenArray = $yt->getFormUploadToken($myVideoEntry, $tokenHandlerUrl);
$tokenValue = $tokenArray['token'];
$postUrl = $tokenArray['url'];
// place to redirect user after upload
$nextUrl = 'http://example.com/your-youtube-upload';
Next create the form to upload a video the action is set to $postUrl and appended $nexturl to this, also make sure to add enctype to the form in order to upload files.
echo '<form action="'. $postUrl .'?nexturl='. $nextUrl .
'" method="post" enctype="multipart/form-data">'.
'<input name="file" type="file"/>'.
'<input name="token" type="hidden" value="'. $tokenValue .'"/>'.
'<input value="Upload Video File" type="submit" />'.
'</form>';
It's aso useful to show a success message on the page this can be done by looking for a GET request of status the value of status if successful will be 200.
if(isset($_GET['status']) && $_GET['status'] == '200'){
echo '<h1>Video Uploaded</h1>';
}
For other response code see this reference: https://developers.google.com/youtube/2.0/reference#Response_codes_uploading_videos
<?php
session_start();
require_once 'Zend/Loader.php'; // the Zend dir must be in your include_path
Zend_Loader::loadClass('Zend_Gdata_YouTube');
Zend_Loader::loadClass('Zend_Gdata_AuthSub');
function getAuthSubRequestUrl()
{
$next = 'http://dcblog.dev/demos/youtube-upload';
$scope = 'http://gdata.youtube.com';
$secure = false;
$session = true;
return Zend_Gdata_AuthSub::getAuthSubTokenUri($next, $scope, $secure, $session);
}
//generate link if no session or token has been requested
if (!isset($_SESSION['sessionToken']) && !isset($_GET['token']) ){
echo '<a href="' . getAuthSubRequestUrl() . '">Login to YouTube API!</a>';
//if token has been requested but not saved to a session then save the new token to a session
} else if (!isset($_SESSION['sessionToken']) && isset($_GET['token'])) {
$_SESSION['sessionToken'] = Zend_Gdata_AuthSub::getAuthSubSessionToken($_GET['token']);
}
if(isset($_SESSION['sessionToken'])) {
//authenticat with youtube
$httpClient = Zend_Gdata_AuthSub::getHttpClient($_SESSION['sessionToken']);
$developerKey = 'you-developer-key-here';
$applicationId = 'Demo Application';
$yt = new Zend_Gdata_YouTube($httpClient, $applicationId, NULL, $developerKey);
// create a new VideoEntry object
$myVideoEntry = new Zend_Gdata_YouTube_VideoEntry();
$myVideoEntry->setVideoTitle('My Test Movie');
$myVideoEntry->setVideoDescription('My Test Movie');
$myVideoEntry->setVideoCategory('Autos'); // The category must be a valid YouTube category!
$tokenHandlerUrl = 'http://gdata.youtube.com/action/GetUploadToken';
$tokenArray = $yt->getFormUploadToken($myVideoEntry, $tokenHandlerUrl);
$tokenValue = $tokenArray['token'];
$postUrl = $tokenArray['url'];
// place to redirect user after upload
$nextUrl = 'http://example.com/your-youtube-upload';
// build the form
echo '<form action="'. $postUrl .'?nexturl='. $nextUrl .
'" method="post" enctype="multipart/form-data">'.
'<input name="file" type="file"/>'.
'<input name="token" type="hidden" value="'. $tokenValue .'"/>'.
'<input value="Upload Video File" type="submit" />'.
'</form>';
if(isset($_GET['status']) && $_GET['status'] == '200'){
echo '<h1>Video Uploaded</h1>';
}
}
Subscribe to my newsletter for the latest updates on my books and digital products.
Find posts, tutorials, and resources quickly.
Subscribe to my newsletter for the latest updates on my books and digital products.